由於seminar與論文寫作的需要,得先建構好LaTex的環境,而原本的LaTex是不支援中文字的,需要額外package (package名稱CJK ,代表 Chinese、Japanese、Korean)才行,這篇文章就是紀錄解決LaTex處理中文的問題。
以Windows為例,安裝
MikTex (過程可以參考
這則PDF,但是中文字我不是用他提到的方法解決,後面會說)
安裝完成之後,先把MikTex的套件庫進行同步更新,在開始功能表的搜尋功能,尋找"Miktex Package Manager (Admin)",並且選擇"Repository"-->"Synchronize"
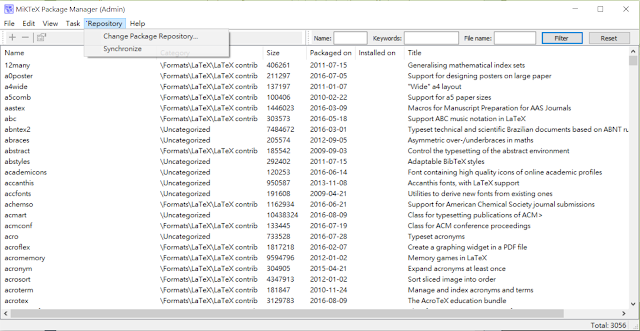 |
MikText Package Manager |
裝好MikTex之後,基本上他會給你一個叫做Texworks的編輯器,不過介面有點陽春
所以我選擇安裝另外的編輯器
Texmaker 來用
(MikTex跟Texmaker像是compiler跟IDE的關係,意即你可以選擇自己喜歡的editor來用 [註1])
接著打開Texmaker,左邊區域是檔案架構,中間是編輯區,右邊是編譯後預覽PDF
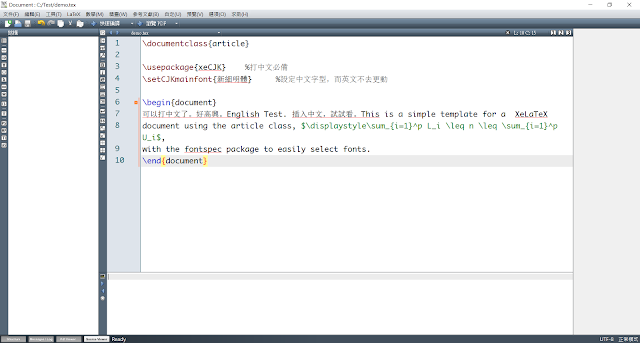 |
Texmaker執行畫面 |
新增一個文件,並且copy-paste底下取自ptt LaTex版的範例 [註2]
\documentclass{article}
\usepackage{xeCJK} %打中文必備
\setCJKmainfont{新細明體} %設定中文字型,而英文不去更動
\begin{document}
可以打中文了。好高興。English Test. 插入中文,試試看。This is a simple template for a XeLaTeX
document using the article class, $\displaystyle\sum_{i=1}^p L_i \leq n \leq \sum_{i=1}^p U_i$,
with the fontspec package to easily select fonts.
\end{document}
之後點"快速編譯"左邊的箭頭來compile,或者使用快捷鍵F1
此時系統應該會提示有缺少的package,全部按yes讓他去下載安裝,輪到xetex-def的package時,會無法下載,錯誤訊息寫"xetex-def not found"
Google後得知xetex-def已經被捨棄,改用graphics-def取代 [註3]
故需打開MikText Package Manager (Admin),手動找尋graphics-def來安裝
裝好回到Texmaker按F1,就能順利compile成功!!
順便附上爬文時找到一些教學介紹
大家來學LaTex
http://web.math.isu.edu.tw/yeh/HowTo/HowToTex/latex123.pdf (雖然是2004的文件,不過應該是基礎入門的經典了,原本網站已經連不上,這份PDF是在義守大學網頁找到的)
XeTeX:解決 LaTeX 惱人的中文字型問題:
http://www.hitripod.com/blog/2011/04/xetex-chinese-font-cjk-latex/
Miktex+texstudio支持中文内容的方法
https://yinqingwang.wordpress.com/2015/06/10/miktex%2Btexstudio%E6%94%AF%E6%8C%81%E4%B8%AD%E6%96%87%E5%86%85%E5%AE%B9%E7%9A%84%E6%96%B9%E6%B3%95/
[註1] 關於LaTex的介紹與版本比較可以參考
LaTex的各種發行板與編譯器的比較,裡面表列了常見的compiler與editor的比較,如果你喜歡,也可以用純文字編輯器Vim、Notepad++打完透過command line下指令compile
[註2]
https://www.ptt.cc/bbs/LaTeX/M.1366782607.A.202.html
[註3]
http://tex.stackexchange.com/questions/319150/miktex-xetex-def-package-installation